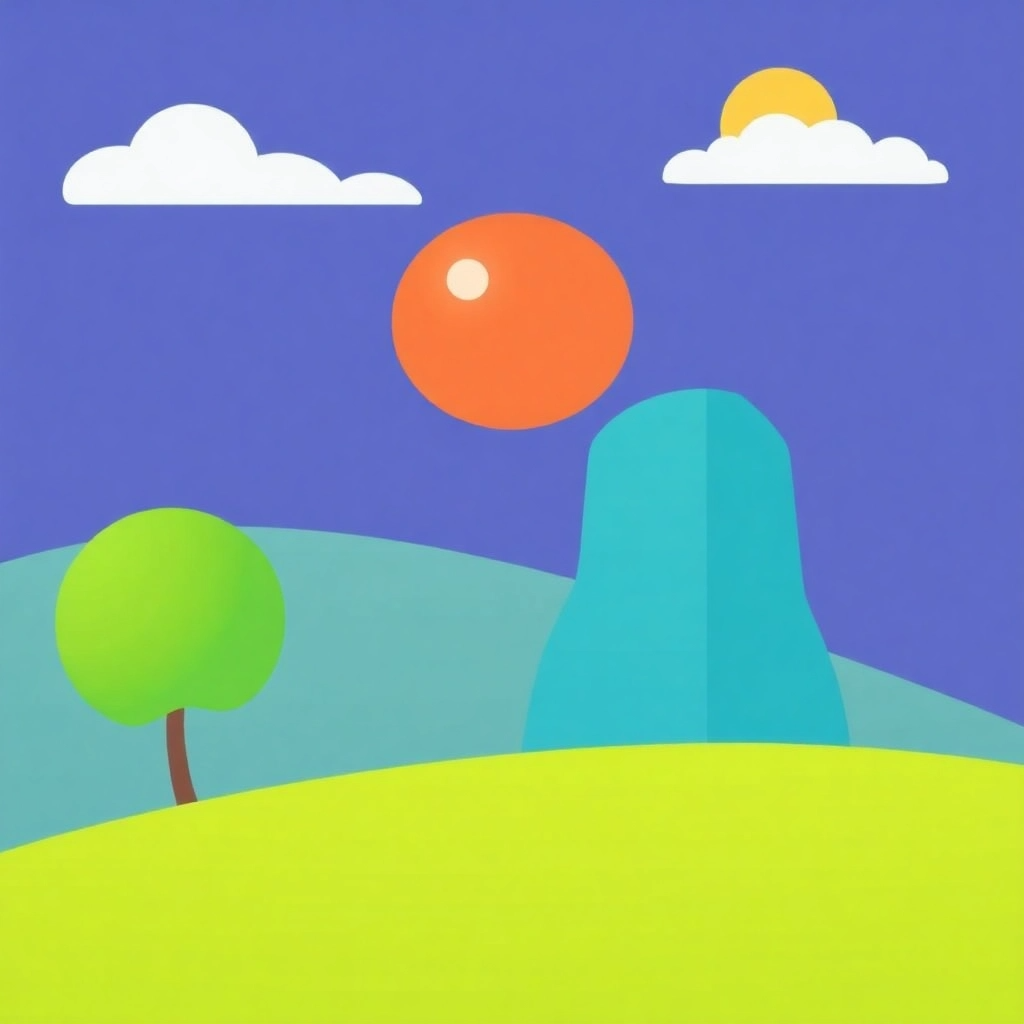
CSS Houdini: The Future of CSS
For years, CSS has been a powerful styling language, but its capabilities have been largely defined by browser vendors. Developers could only use the features implemented in browsers, often waiting years for new proposals to become standardized and widely available. CSS Houdini changes this paradigm by exposing low-level APIs that allow developers to extend CSS itself, creating new styling and layout possibilities directly within the browser’s rendering engine.
What is CSS Houdini and Why It Matters
Houdini is a collection of APIs that give developers direct access to the CSS Object Model (CSSOM), enabling them to write code that the browser can parse as CSS, thereby creating new CSS features without waiting for native browser implementation. Think of it as bringing the extensibility of JavaScript to the world of CSS rendering.
Why does this matter?
- Innovation: Developers can experiment with and implement new CSS features, pushing the boundaries of web design faster than browser vendors alone.
- Performance: Houdini APIs often run in a separate rendering process (the rendering worklet), meaning custom CSS features can perform better than JavaScript-based polyfills that manipulate the DOM.
- Polyfills: Houdini allows for the creation of high-performance polyfills for new or experimental CSS features, making them usable sooner.
- Customization: Developers can create highly customized styling and layout behaviors tailored to specific project needs.
The Houdini APIs
Houdini consists of several distinct APIs, each targeting a different part of the CSS rendering pipeline:
1. CSS Properties and Values API
This API allows developers to define custom CSS properties with specific types, initial values, and inheritance behavior. This makes custom properties more robust and animatable.
javascript
// Register a custom property
CSS.registerProperty({
name: '--my-color',
syntax: '<color>',
inherits: false,
initialValue: 'black'
});
CSS.registerProperty({
name: '--my-length',
syntax: '<length-percentage>',
inherits: true,
initialValue: '0px'
});
css
.element {
--my-color: blue;
--my-length: 100px;
background-color: var(--my-color);
width: var(--my-length);
/* Now animatable because the type is known */
transition: --my-color 0.5s, --my-length 0.5s;
}
.element:hover {
--my-color: red;
--my-length: 200px;
}
2. CSS Typed OM (Object Model)
This API provides a more structured and performant way to interact with CSS values in JavaScript, replacing string-based manipulation with typed objects.
javascript
// Old way (string-based)
// element.style.opacity = '0.5';
// const opacity = parseFloat(element.style.opacity);
// New way (Typed OM)
const element = document.querySelector('.my-element');
// Set value
element.attributeStyleMap.set('opacity', CSS.number(0.5));
// Get value
const opacityValue = element.attributeStyleMap.get('opacity'); // Returns a CSSUnitValue object
console.log(opacityValue.value); // 0.5
// Set complex value
element.computedStyleMap().get('margin-top'); // Returns CSSUnitValue { value: 10, unit: 'px' }
3. CSS Painting API (Paint Worklet)
This is one of the most visually impactful Houdini APIs. It allows developers to create custom drawing functions using JavaScript that can be used directly in CSS properties like background-image
, border-image
, or mask-image
.
javascript
// paintworklet.js
registerPaint('checkerboard', class {
paint(ctx, size) {
const squareSize = 10;
for (let y = 0; y < size.height / squareSize; y++) {
for (let x = 0; x < size.width / squareSize; x++) {
ctx.fillStyle = (x + y) % 2 === 0 ? 'black' : 'white';
ctx.fillRect(x * squareSize, y * squareSize, squareSize, squareSize);
}
}
}
});
javascript
// Main thread: Register the worklet
CSS.paintWorklet.addModule('paintworklet.js');
css
.element {
background-image: paint(checkerboard);
}
This enables creating custom patterns, gradients, borders, and other visual effects programmatically and performantly.
4. CSS Layout API (Layout Worklet)
This API allows developers to define custom layout algorithms, essentially creating their own display
modes (like display: masonry;
or display: centered-grid;
).
javascript
// layoutworklet.js
registerLayout('masonry', class {
async layout(children, edges, constraints, styleMap) {
// ... complex layout logic ...
// Calculate positions and sizes for children
// Return { autoBlockSize, childFragments }
}
});
javascript
// Main thread: Register the worklet
CSS.layoutWorklet.addModule('layoutworklet.js');
css
.container {
display: layout(masonry);
}
The Layout API is powerful but complex, offering deep control over how elements are positioned and sized.
5. CSS Animation Worklet
This API aims to provide high-performance, imperative animations that run off the main thread, similar to the Web Animations API but with more control and potentially better performance for complex scenarios.
Browser Support and Future
Browser support for Houdini APIs varies:
- Properties and Values API: Good support across modern browsers.
- Typed OM: Good support.
- Paint API: Good support.
- Layout API: Limited support (mostly Chrome/Edge behind flags).
- Animation Worklet: Limited support (mostly Chrome/Edge behind flags).
While some APIs like Layout and Animation Worklet are still maturing, the Properties and Values, Typed OM, and Paint APIs are already usable in production environments, offering significant benefits.
Houdini represents a fundamental shift in CSS development, empowering developers to extend the language itself. As support grows, expect to see more innovative and performant styling and layout techniques emerge, driven directly by the web development community.